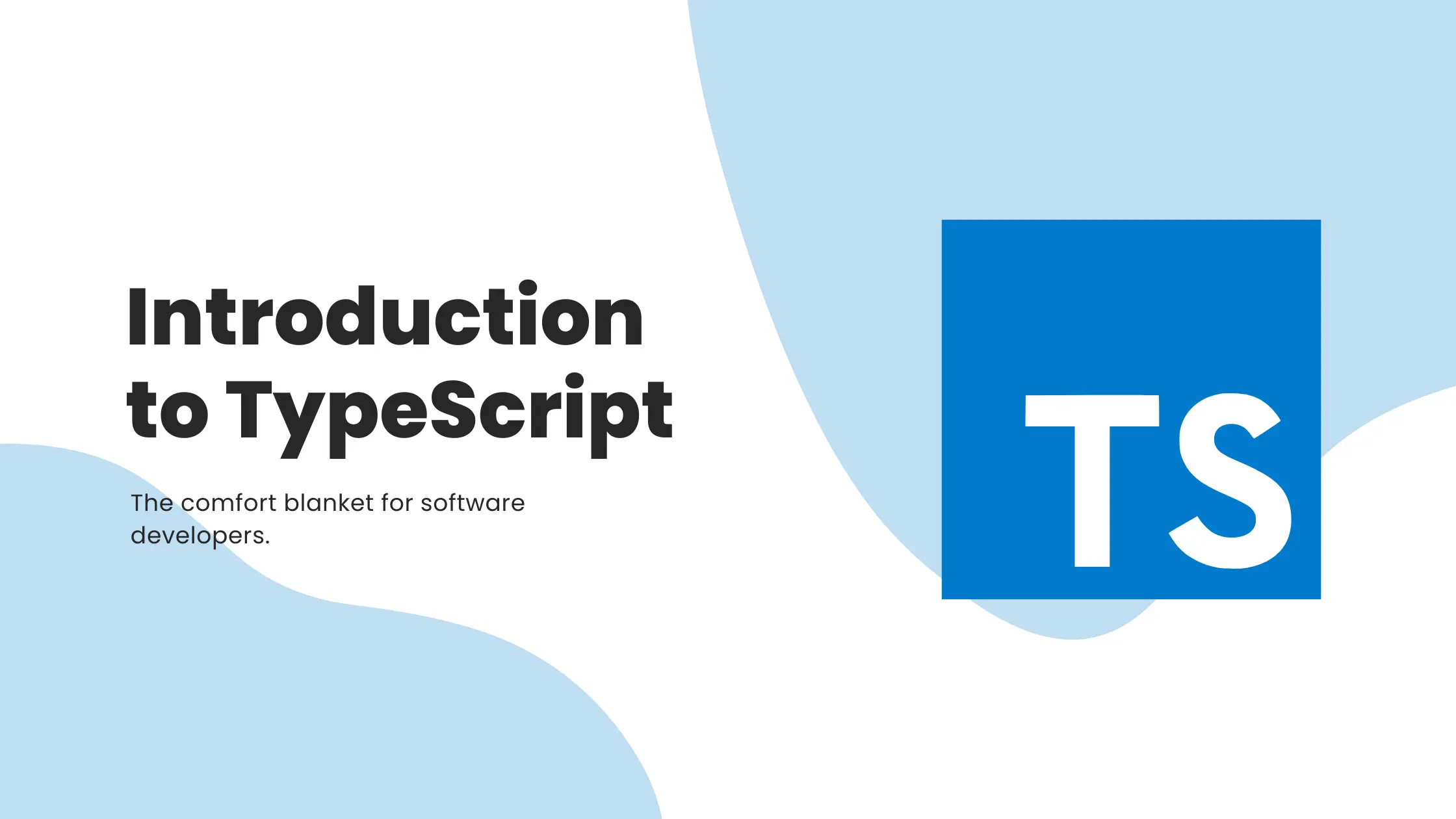
An Introduction to TypeScript
Learn the essential basics of TypeScript and its significant advantages over traditional JavaScript.
An Introduction to TypeScript
TypeScript is a powerful superset of JavaScript that adds static types. It is designed to improve the development process by providing better tooling, enhanced code quality, and reduced runtime errors. In this article, we'll cover the essential basics of TypeScript and its significant advantages over traditional JavaScript.
1. What is TypeScript?
TypeScript is an open-source programming language developed by Microsoft. It builds on JavaScript by adding static type definitions, allowing developers to specify the types of variables, function parameters, and return values. This feature helps catch errors during development, making the code more robust and maintainable.
2. Key Features of TypeScript
Strong Typing
One of the primary advantages of TypeScript is its strong typing system. By explicitly defining types, you can catch potential errors before they occur at runtime. For example:
function greet(name: string): string {
return `Hello, ${name}!`;
}
console.log(greet('Jane')); // Valid
console.log(greet(42)); // Error: Argument of type 'number' is not assignable to parameter of type 'string'.
Interfaces
TypeScript allows you to define interfaces, which are contracts for the structure of objects. This helps enforce consistency and improves code readability.
interface User {
id: number;
name: string;
}
const user: User = {
id: 1,
name: 'Jane Smith',
};
Type Inference
TypeScript is smart enough to infer types based on the values assigned to variables, reducing the need for explicit type annotations in many cases:
let count = 10;
3. Advantages of Using TypeScript
- Early Error Detection: TypeScript catches errors at compile time, preventing many common runtime errors.
- Improved Code Readability: The use of types and interfaces makes the code easier to understand and maintain.
- Enhanced IDE Support: Many modern IDEs provide better autocompletion and navigation features when working with TypeScript.
4. Getting Started with TypeScript
To start using TypeScript, you need to install it globally via npm:
npm install -g typescript
Once installed, you can create a TypeScript file (with a .ts
extension) and compile it to JavaScript using the TypeScript compiler:
tsc filename.ts
Conclusion
TypeScript is a powerful tool for modern web development, enhancing JavaScript with strong typing and better tooling. By understanding its key features and advantages, you can leverage TypeScript to write more robust and maintainable code. Whether you're building a small project or a large-scale application, TypeScript can significantly improve your development experience.