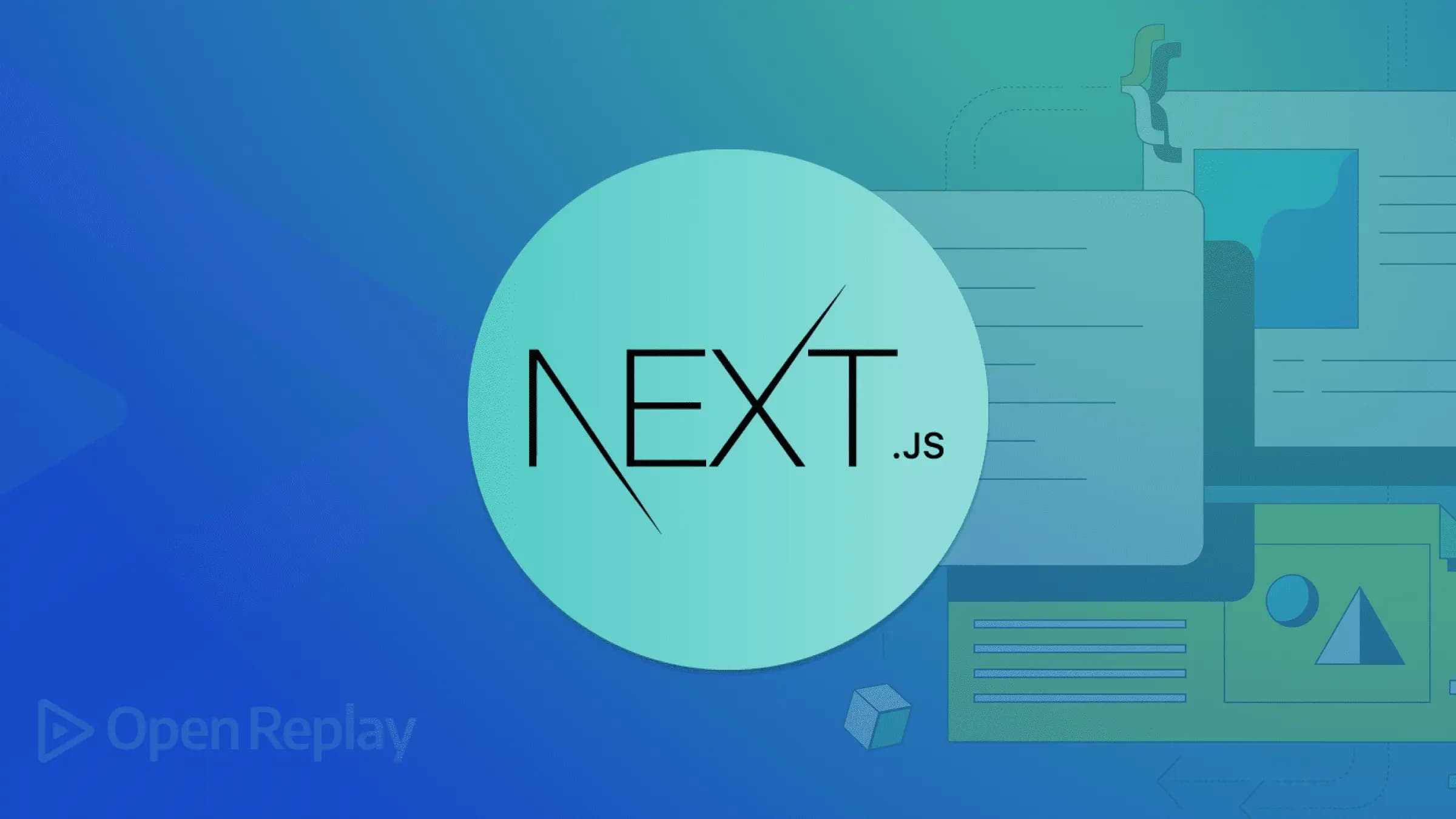
Deploying a Next.js Application
Follow these essential steps to successfully deploy your Next.js application with ease.
Deploying a Next.js application is straightforward, thanks to its optimized build system and support for various hosting providers. In this guide, we’ll cover the main steps and strategies for a smooth deployment, from static generation to server-rendering and environment configuration.
1. Preparing Your Application for Deployment
Before deploying, ensure your application is optimized by building it for production. Run the following command to build the project:
npm run build
This creates a .next
folder containing your optimized application. This step is crucial for both static and dynamic pages, as it applies tree-shaking and code-splitting to reduce load times.
2. Static Generation with getStaticProps
Static Site Generation (SSG) is a recommended strategy for Next.js apps with content that doesn’t change frequently. SSG pre-renders pages at build time, making it ideal for pages like blogs and landing pages.
Use getStaticProps
to generate pages statically:
export async function getStaticProps() {
const data = await fetchData();
return {
props: { data },
};
}
3. Server-side Rendering (SSR) with getServerSideProps
For pages that require up-to-date data on every request, use Server-side Rendering (SSR) with getServerSideProps
. This approach is suitable for pages displaying frequently changing data.
export async function getServerSideProps() {
const data = await fetchData();
return {
props: { data },
};
}
When deploying, SSR pages are generated at request time, making them slower than SSG but beneficial for dynamic content.
4. API Routes in Next.js
Next.js lets you create API routes by adding files to the pages/api
directory. API routes are server-side functions that can fetch data, process requests, or interact with external services.
// pages/api/hello.js
export default function handler(req, res) {
res.status(200).json({ message: 'Hello from Next.js!' });
}
These routes are ideal for handling form submissions, fetching data, and proxying requests, and they’re automatically deployed as serverless functions.
5. Using Environment Variables
For secure deployments, use environment variables to store sensitive information like API keys. Next.js allows you to define variables in a .env.local
file, which are accessible via process.env
.
Use these variables in your code as follows:
const apiUrl = process.env.NEXT_PUBLIC_API_URL;
6. Choosing a Deployment Platform
You can deploy Next.js applications to various platforms. Below are some popular options:
- Vercel: The official platform for Next.js with features like previews, automatic builds, and serverless functions.
- Netlify: Another popular platform that supports static generation and serverless functions.
- AWS Amplify and Azure Static Web Apps: Provide extensive scaling and backend support, ideal for large applications.
To deploy with Vercel, simply run:
vercel
7. Using Fallbacks for Dynamic Routes
When using SSG with dynamic pages, sometimes not all routes are known at build time. Set fallback: true
to dynamically generate missing pages on the first request.
export async function getStaticPaths() {
return {
paths: [], // Specify known paths
fallback: true,
};
}
8. Performance Optimizations
To further optimize performance, consider:
- Lazy-loading images: Use the
next/image
component for automatic image optimization. - Code splitting: Next.js automatically splits code, loading only the necessary files for each page.
- Cache-Control headers: Configure caching headers for assets in
next.config.js
.
Conclusion
Deploying a Next.js application is seamless thanks to its support for SSG, SSR, and API routes. With these strategies, your application will be optimized, scalable, and ready to handle production-level traffic. Whether you choose Vercel, Netlify, or another platform, Next.js makes deployment efficient and straightforward.