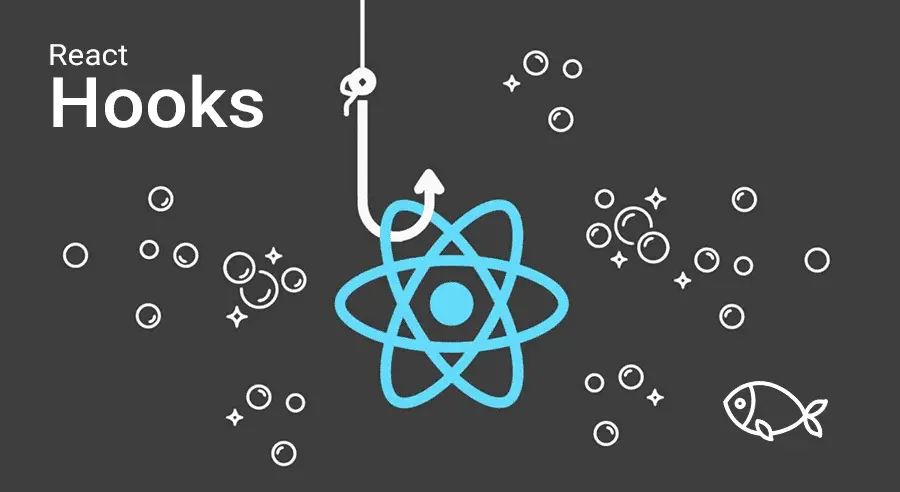
Understanding React Hooks
React hooks are functions that let you use state and other React features in functional components. Learn about the useState and useEffect hooks and how they simplify state management and side effects in React applications.
React hooks are functions that let you use state and other React features in functional components. Hooks simplify the way we handle state, side effects, and other functionalities, making functional components more powerful.
1. useState
The useState hook allows you to add state to functional components. It returns a state variable and a function to update it.
const [count, setCount] = useState(0);
- count: The current value of the state.
- setCount: A function to update the value of `count`.
This hook is ideal when managing values like form inputs, counters, or any data that can change.
2. useEffect
The useEffect hook lets you perform side effects in functional components, such as:
- Fetching data from an API.
- Manually changing the DOM.
- Setting up subscriptions.
It runs after the first render and after every update, by default.
useEffect(() => {
// Code to run after the component renders or updates
}, []);
- The dependency array controls when the side effect is executed.
- Passing an empty array (`[]`) means it runs only after the initial render.
Hooks like useState and useEffect help bring simplicity and flexibility to your code, replacing the need for class components in many situations.
Benefits of Using Hooks
- Simplifies State Management: You can add and manage component state with less boilerplate.
- Code Reusability: Extract reusable logic into custom hooks.
- Functional Components: All components can be functional, which simplifies the component structure.
React hooks have become an essential part of the React ecosystem, providing developers with easier, more readable, and more maintainable code.