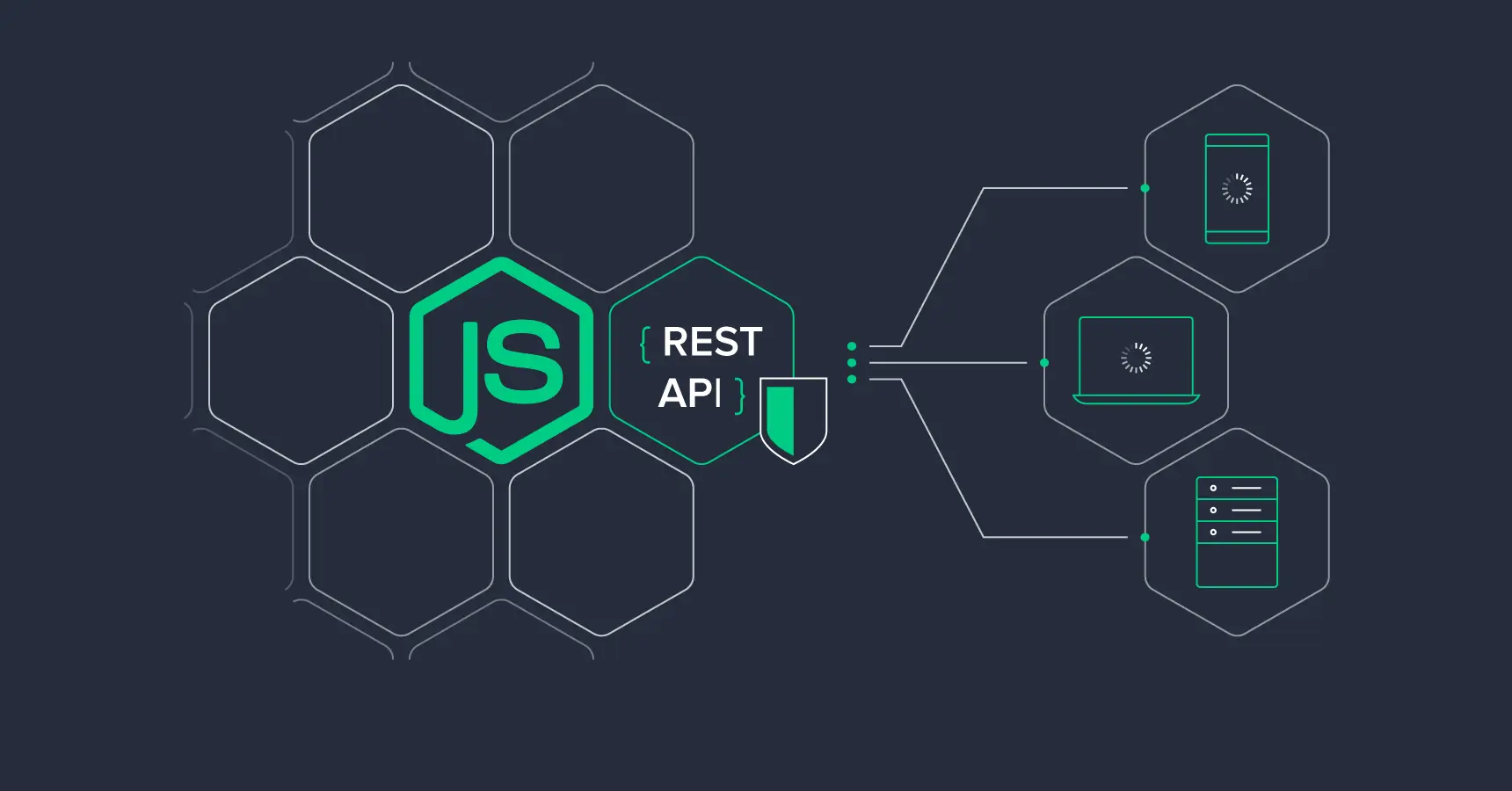
Building RESTful APIs with Node.js
Comprehensive guide on creating RESTful APIs using Node.js and the Express framework effectively.
Building RESTful APIs with Node.js and Express
RESTful APIs are a fundamental part of modern web development. They provide a way for different services and systems to communicate over the web. In this guide, we’ll walk through how to create a RESTful API using Node.js and the Express framework.
1. Setting Up Node.js and Express
To begin building a RESTful API, you need to have Node.js installed. Express is a lightweight and flexible framework that helps in building APIs quickly. You can install it via npm:
npm install express
2. Creating the Basic Express App
Once Express is installed, you can create a basic Express app that will serve as the backbone of your API:
const express = require('express');
const app = express();
const PORT = process.env.PORT || 3000;
app.get('/', (req, res) => {
res.send('Welcome to our RESTful API');
});
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
In this code, we define a simple GET request that responds with a message. The app listens on port 3000 or whatever port is set in your environment.
3. Building CRUD Operations
RESTful APIs typically allow for four basic operations, known as CRUD: Create, Read, Update, and Delete. Here’s how you can implement each operation in your Express app:
Creating Data (POST)
To handle POST requests for creating new data, you can use the following:
app.use(express.json());
app.post('/api/items', (req, res) => {
const newItem = req.body;
// Save the new item to your database (mocked here)
res.status(201).send(newItem);
});
Reading Data (GET)
You can use GET requests to retrieve data:
app.get('/api/items', (req, res) => {
// Fetch data from the database (mocked here)
const items = [{ id: 1, name: 'Item 1' }];
res.send(items);
});
Updating Data (PUT)
For updating an existing resource, you can use PUT:
app.put('/api/items/:id', (req, res) => {
const { id } = req.params;
const updatedItem = req.body;
// Update the item in your database (mocked here)
res.send({ id, ...updatedItem });
});
Deleting Data (DELETE)
To delete data, you can implement the DELETE request:
app.delete('/api/items/:id', (req, res) => {
const { id } = req.params;
// Remove the item from your database (mocked here)
res.status(204).send();
});
4. Middleware in Express
Middleware functions are functions that have access to the request object (req
), the response object (res
), and the next middleware function in the application’s request-response cycle. For example, you can log requests to the console:
app.use((req, res, next) => {
console.log(`${req.method} request to ${req.url}`);
next();
});
5. Error Handling
Good error handling is crucial in a RESTful API. You can create a middleware to handle errors:
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something went wrong!');
});
Conclusion
Building a RESTful API with Node.js and Express is straightforward and efficient. By setting up routes and handling CRUD operations, you can create an API that powers modern web applications. With tools like Express, you can scale your API and integrate additional features such as authentication, database connections, and more.